//============================================================================
// Name : Assignment.cpp
// Description : Ask the users to enter the width and length of a rectangle then // : outputs the area
// : contains functions getLength, getWidth, and getArea
// Status : Complete
//============================================================================
#include <iostream>
using namespace std;
/*
* 5A:
* Rectangle Area – Complete the Program
* The Student CD contains a partially written program named AreaRectangle.cpp
* Your job is to complete the program. When it is complete, the program will
* ask the user to enter the width and length of a rectangle, and then display
* the rectangle’s area. The program calls the following functions, which have not
* been written:
* getLenght – This function should ask the user to enter the rectangle’s length,
* and then return the value as a double
* getWidth – This function should ask the user to enter the rectangle’s width, and
* then return that value as a double
* getArea – This function should accept the rectangle’s length and width as arguments,
* and return the rectangle’s area. The area is calculated by multiplying the
* length by the width.
* displayData – This function should accept the rectangle’s length, width, and
* area as arguments, and display them in an appropriate message on the screen.
*/
// Function prototypes
double getLength();
double getWidth();
double getArea(double, double);
void displayData(double, double, double);
int main()
{
double length; // To hold the rectangle’s length
double width; // To hold the rectangle’s width
double area; // To hold the rectangle’s area
// Get the rectangle’s length.
length = getLength();
// Get the rectangle’s width.
width = getWidth();
// Get the rectangle’s area.
area = getArea(length, width);
// Display the rectangle’s data.
displayData(length, width, area);
return 0;
}
//***************************************************
// getLength function *
//***************************************************
double getLength()
{
double length; // To hold the length
// Get the length.
cout << “Enter the length: “;
cin >> length;
// Return the length.
return length;
}
//***************************************************
// getWidth function *
//***************************************************
double getWidth()
{
double width; // To hold the width
// Get the width.
cout << “Enter the width: “;
cin >> width;
// Return the width.
return width;
}
//***************************************************
// getArea function *
//***************************************************
double getArea(double length, double width)
{
// Return the area.
return length * width;
}
//***************************************************
// displayData function *
//***************************************************
void displayData(double length, double width, double area)
{
cout << “\nRectangle Data\n”
<< “————–\n”
<< “Length: ” << length << endl
<< “Width: ” << width << endl
<< “Area: ” << area << endl;
}
Output:
5b:
//============================================================================
// Name : Assignment5b.cpp
// Author : ZackMaher
// Version :
// Date : 10/04/2020
// Description : Displays the distance in meters of a falling object from 1 to 10 seconds
// Status : Complete
//============================================================================
#include <iostream>
#include <cmath>
using namespace std;
/*
* 5B:
* Falling Distance
* When an object is falling because of gravity, the following formula can be
* used to determine distance the object falls in a specific time period:
* d = 1/2 gt squared
* The variables in the formula are as follows: d is the distance in meters,
* g is 9.8, and t is the amount of time, in seconds, that the object has been falling
* Write a function named fallingDistance that accepts an object’s falling time (in seconds)
* as an argument. The function should return the distance, in meters, that the object has fallen
* during that time interval. Write a program that demonstrates the function by calling it in a loop
* that passes the values 1 through 10 as arguments, and displays the return value
*/
/*
In function main:
Write a For loop that runs from 1 to 10.
Inside the loop:
*/
/*
1. Call the distance function and send it the loop counter as an argument. Notice that the
loop counter will take the values 1 (second) 2(seconds) ……10(seconds); basically you
are using the loop counter to pass the time in seconds starting from 1 to 10.
2. Receive the distance (as double) from distance function
3. Display the time and the falling distance
4. Distance function:
1. Receives time in seconds ( integer)
2. Calculates the distance and returns it
You may need to declare a local variable for the distance
You need to implement the formula for calculating the distance as given in the
assignment specification
*/
//function prototype fallingDistance that returns a double of distance in meters that take an int variable seconds
double fallingDistance(int);
int main() {
int seconds = 1;
for(seconds = 1; seconds<=10; seconds++){
fallingDistance(seconds);
}
return 0;
}
double fallingDistance(int seconds){
double distanceInMeters=0.0;
distanceInMeters = .5*9.8*pow(seconds,2);
cout << “Seconds: ” << seconds << ” seconds | Distance In Meters: ” << distanceInMeters << ” Meters” << endl;
return distanceInMeters;
}
Output:
5c:
//============================================================================
// Name : Assignment 5c.cpp
// Author : Zack Maher
// Date : 10/4/2020
// Description : inputs score 5 times, removes the lowest score, and outputs the
// : average score
// Status : Complete
//============================================================================
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
/*
* 5C:
* Lowest Score Drop
* Write a program that calculates the average of a group of test scores, where the lowest
* score in the group is dropped. It should use the following functions:
* void getScore() should ask the user for a test score, store it in a reference parameter variable,
* and validate it. This function should be called by main once for each of the five scores to be entered.
* void calcAverage() should calculate and display the average of the four highest scores. This function should be called
* just once by main, and should be passed the five scores.
* int findLowest() should find and return the lowest of the five scores passed to it. It should be called by calcAverage,
* which uses the function to determine which of the five scores to drop.
* Input validation: Do not accept test scores lower than 0 or higher than 100.
*/
//function prototype getScore returns type void take a parameters of double by reference
void getScore(int&,vector<int>&);
//function prototype calcAverage() returns type void;
void calcAverage(int&, int&, int&, vector<int>&, vector<int>&);
//function prototype findLowerst returns type double;
int findLowest(vector<int>&);
int main() {
vector<int> allScores;
vector<int> allScoresDroppedOne;
int thisScore =0;
int scoreAverage=0;
int sumOfScoresDroppedOne=0;
int lowestScore=0;
getScore(thisScore, allScores);
calcAverage(scoreAverage, lowestScore, sumOfScoresDroppedOne, allScores, allScoresDroppedOne);
return 0;
}
void getScore(int& thisScore,vector<int>& allScores){
int count=0;
//Input validation that this score is between 0 and 100
while(count < 5){
cout<<“Enter a test score between 0 and 100” << endl;
cin >> thisScore;
if(thisScore < 0 || thisScore > 100){
cout << “Invalid input, please try again”<<endl;
cout << endl;
}
else{
cout << “Current Count: ” << count << ” Score: ” << thisScore << endl;
allScores.push_back(thisScore);
count++;
cout << endl;
}
}
}
void calcAverage(int& scoreAverage, int& lowestScore, int& sumOfScoresDroppedOne, vector<int>& allScores, vector<int>& allScoresDroppedOne){
lowestScore = findLowest(allScores);
cout << “These are the scores before the lowest score is dropped: ” << endl;
for(int a=0; a < allScores.size(); a++){
cout << allScores.at(a) << ‘ ‘;
if(allScores.at(a) != lowestScore){
allScoresDroppedOne.push_back(allScores.at(a));
}
}
int droppedScoresSize = allScoresDroppedOne.size();
cout << “\nThese are the scores after lowest score is dropped: ” << endl;
for(int i=0; i < allScoresDroppedOne.size(); i++){
cout << allScoresDroppedOne.at(i) << ‘ ‘;
sumOfScoresDroppedOne = sumOfScoresDroppedOne + allScoresDroppedOne.at(i);
}
scoreAverage = sumOfScoresDroppedOne / droppedScoresSize;
cout << endl;
cout << “scoreAverage is: ” << scoreAverage << endl;
}
int findLowest(vector<int>& allScores){
int localLowest = *min_element(allScores.begin(), allScores.end());
cout << “Lowest Score is : ” << localLowest << endl;
return localLowest;
}
//============================================================================
// Name : Assignment.cpp
// Description : Ask the users to enter the width and length of a rectangle then // : outputs the area
// : contains functions getLength, getWidth, and getArea
// Status : Complete
//============================================================================
#include <iostream>
using namespace std;
/*
* 5A:
* Rectangle Area – Complete the Program
* The Student CD contains a partially written program named AreaRectangle.cpp
* Your job is to complete the program. When it is complete, the program will
* ask the user to enter the width and length of a rectangle, and then display
* the rectangle’s area. The program calls the following functions, which have not
* been written:
* getLenght – This function should ask the user to enter the rectangle’s length,
* and then return the value as a double
* getWidth – This function should ask the user to enter the rectangle’s width, and
* then return that value as a double
* getArea – This function should accept the rectangle’s length and width as arguments,
* and return the rectangle’s area. The area is calculated by multiplying the
* length by the width.
* displayData – This function should accept the rectangle’s length, width, and
* area as arguments, and display them in an appropriate message on the screen.
*/
// Function prototypes
double getLength();
double getWidth();
double getArea(double, double);
void displayData(double, double, double);
int main()
{
double length; // To hold the rectangle’s length
double width; // To hold the rectangle’s width
double area; // To hold the rectangle’s area
// Get the rectangle’s length.
length = getLength();
// Get the rectangle’s width.
width = getWidth();
// Get the rectangle’s area.
area = getArea(length, width);
// Display the rectangle’s data.
displayData(length, width, area);
return 0;
}
//***************************************************
// getLength function *
//***************************************************
double getLength()
{
double length; // To hold the length
// Get the length.
cout << “Enter the length: “;
cin >> length;
// Return the length.
return length;
}
//***************************************************
// getWidth function *
//***************************************************
double getWidth()
{
double width; // To hold the width
// Get the width.
cout << “Enter the width: “;
cin >> width;
// Return the width.
return width;
}
//***************************************************
// getArea function *
//***************************************************
double getArea(double length, double width)
{
// Return the area.
return length * width;
}
//***************************************************
// displayData function *
//***************************************************
void displayData(double length, double width, double area)
{
cout << “\nRectangle Data\n”
<< “————–\n”
<< “Length: ” << length << endl
<< “Width: ” << width << endl
<< “Area: ” << area << endl;
}
Output:
5b:
//============================================================================
// Name : Assignment5b.cpp
// Author : ZackMaher
// Version :
// Date : 10/04/2020
// Description : Displays the distance in meters of a falling object from 1 to 10 seconds
// Status : Complete
//============================================================================
#include <iostream>
#include <cmath>
using namespace std;
/*
* 5B:
* Falling Distance
* When an object is falling because of gravity, the following formula can be
* used to determine distance the object falls in a specific time period:
* d = 1/2 gt squared
* The variables in the formula are as follows: d is the distance in meters,
* g is 9.8, and t is the amount of time, in seconds, that the object has been falling
* Write a function named fallingDistance that accepts an object’s falling time (in seconds)
* as an argument. The function should return the distance, in meters, that the object has fallen
* during that time interval. Write a program that demonstrates the function by calling it in a loop
* that passes the values 1 through 10 as arguments, and displays the return value
*/
/*
In function main:
Write a For loop that runs from 1 to 10.
Inside the loop:
*/
/*
1. Call the distance function and send it the loop counter as an argument. Notice that the
loop counter will take the values 1 (second) 2(seconds) ……10(seconds); basically you
are using the loop counter to pass the time in seconds starting from 1 to 10.
2. Receive the distance (as double) from distance function
3. Display the time and the falling distance
4. Distance function:
1. Receives time in seconds ( integer)
2. Calculates the distance and returns it
You may need to declare a local variable for the distance
You need to implement the formula for calculating the distance as given in the
assignment specification
*/
//function prototype fallingDistance that returns a double of distance in meters that take an int variable seconds
double fallingDistance(int);
int main() {
int seconds = 1;
for(seconds = 1; seconds<=10; seconds++){
fallingDistance(seconds);
}
return 0;
}
double fallingDistance(int seconds){
double distanceInMeters=0.0;
distanceInMeters = .5*9.8*pow(seconds,2);
cout << “Seconds: ” << seconds << ” seconds | Distance In Meters: ” << distanceInMeters << ” Meters” << endl;
return distanceInMeters;
}
Output:
5c:
//============================================================================
// Name : Assignment 5c.cpp
// Author : Zack Maher
// Date : 10/4/2020
// Description : inputs score 5 times, removes the lowest score, and outputs the
// : average score
// Status : Complete
//============================================================================
#include <iostream>
#include <algorithm>
#include <vector>
using namespace std;
/*
* 5C:
* Lowest Score Drop
* Write a program that calculates the average of a group of test scores, where the lowest
* score in the group is dropped. It should use the following functions:
* void getScore() should ask the user for a test score, store it in a reference parameter variable,
* and validate it. This function should be called by main once for each of the five scores to be entered.
* void calcAverage() should calculate and display the average of the four highest scores. This function should be called
* just once by main, and should be passed the five scores.
* int findLowest() should find and return the lowest of the five scores passed to it. It should be called by calcAverage,
* which uses the function to determine which of the five scores to drop.
* Input validation: Do not accept test scores lower than 0 or higher than 100.
*/
//function prototype getScore returns type void take a parameters of double by reference
void getScore(int&,vector<int>&);
//function prototype calcAverage() returns type void;
void calcAverage(int&, int&, int&, vector<int>&, vector<int>&);
//function prototype findLowerst returns type double;
int findLowest(vector<int>&);
int main() {
vector<int> allScores;
vector<int> allScoresDroppedOne;
int thisScore =0;
int scoreAverage=0;
int sumOfScoresDroppedOne=0;
int lowestScore=0;
getScore(thisScore, allScores);
calcAverage(scoreAverage, lowestScore, sumOfScoresDroppedOne, allScores, allScoresDroppedOne);
return 0;
}
void getScore(int& thisScore,vector<int>& allScores){
int count=0;
//Input validation that this score is between 0 and 100
while(count < 5){
cout<<“Enter a test score between 0 and 100” << endl;
cin >> thisScore;
if(thisScore < 0 || thisScore > 100){
cout << “Invalid input, please try again”<<endl;
cout << endl;
}
else{
cout << “Current Count: ” << count << ” Score: ” << thisScore << endl;
allScores.push_back(thisScore);
count++;
cout << endl;
}
}
}
void calcAverage(int& scoreAverage, int& lowestScore, int& sumOfScoresDroppedOne, vector<int>& allScores, vector<int>& allScoresDroppedOne){
lowestScore = findLowest(allScores);
cout << “These are the scores before the lowest score is dropped: ” << endl;
for(int a=0; a < allScores.size(); a++){
cout << allScores.at(a) << ‘ ‘;
if(allScores.at(a) != lowestScore){
allScoresDroppedOne.push_back(allScores.at(a));
}
}
int droppedScoresSize = allScoresDroppedOne.size();
cout << “\nThese are the scores after lowest score is dropped: ” << endl;
for(int i=0; i < allScoresDroppedOne.size(); i++){
cout << allScoresDroppedOne.at(i) << ‘ ‘;
sumOfScoresDroppedOne = sumOfScoresDroppedOne + allScoresDroppedOne.at(i);
}
scoreAverage = sumOfScoresDroppedOne / droppedScoresSize;
cout << endl;
cout << “scoreAverage is: ” << scoreAverage << endl;
}
int findLowest(vector<int>& allScores){
int localLowest = *min_element(allScores.begin(), allScores.end());
cout << “Lowest Score is : ” << localLowest << endl;
return localLowest;
}
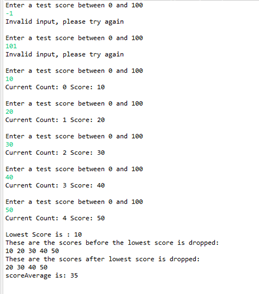