// *******************************************************************************
// Assignment 1: Fortune Teller Program
// Description :
// 1A: Prompts the user for their age, calculates how
// old the user would be in a year, and displays it on the screen.
// 1B: Stores 5 numbers 28, 32, 37, 24, and 33 as double variables,
// sums them, calculates the average
// and prints the average to the screen
// 1C: Outputs the size of char, int, float, and double
// Status : Complete
// Date : August 22, 2020
// ********************************************************************************
#include <iostream>
using namespace std;
int main() {
/*
* 1A Create a project on code blocks.
* Then enter the following program.
* Make sure to indent the block comment but replace your name with mine.
* Also make sure to have proper indentation (use the tab key for indentation)
* and neatly written code
*/
//outputs the assignment # to the screen
cout << “This is Assignment 1A” << endl << endl;
int age;
int new_age;
cout << “Please enter your age::: ” ;
cin >> age;
new_age = age + 1;
cout << “Next year you will be ” << new_age << ” old.\n”;
cout << “Thank you for using my program!” << endl;
/*
* 1B Average of Values
* To get an average of a series of values,
* you add the values up and then divide the sum
* by the number of values.
* Write a program that stores the following values in five variables
* 28, 32, 37, 24, and 33
* the program should first calculate
* the sum of these five variables nad store the result
*in as separate variable named sum.
*Then, the program should divide the sum by 5 to get
* the average. Display the average on the screen
* TIP: Use the double data tyoe for all variables in this program
*/
//outputs the assignment # to the screen
cout << “This is Assignment 1B” << endl << endl;
//store five number variables
double num1 = 28;
double num2 = 32;
double num3 = 37;
double num4 = 24;
double num5 = 33;
//calculate the sum of the five variables and store in a variable called sum
double sum = num1 + num2 + num3 + num4 + num5;
double thisAverage = sum / 5;
cout << “thisAverage: ” << thisAverage << endl;
/*
* 1C Cyborg Data Type Sizes
* You have been given a job as a programmer on a Cyborg supercomputer.
* In order to accomplish some calculations,
* you need to know how many bytes the
* following data types use: char, int, float, and double.
* You do not have any manuals
* so you can’t look this information up.
* Write a C++ program that will determine the
* amount of memory used by these types
* and display the information to the screen.
*/
//outputs the assignment # to the screen
cout << “This is Assignment 1C” << endl << endl;
//size of char
cout << “Size of char: ” << sizeof(char) << endl;
//size of int
cout << “Size of int: ” << sizeof(int) << endl;
//size of float
cout << “Size of float: ” << sizeof(float) << endl;
//size of double
cout << “Size of double: ” << sizeof(double) << endl;
return 0;
}
SCREENSHOT OF OUTPUT:
Please note this screenshot contains output of 1A, 1B, and 1C
// *******************************************************************************
// Assignment 1: Fortune Teller Program
// Author : Zack Maher
// Description :
// 1A: Prompts the user for their age, calculates how
// old the user would be in a year, and displays it on the screen.
// 1B: Stores 5 numbers 28, 32, 37, 24, and 33 as double variables,
// sums them, calculates the average
// and prints the average to the screen
// 1C: Outputs the size of char, int, float, and double
// Status : Complete
// Date : August 22, 2020
// ********************************************************************************
#include <iostream>
using namespace std;
int main() {
/*
* 1A Create a project on code blocks.
* Then enter the following program.
* Make sure to indent the block comment but replace your name with mine.
* Also make sure to have proper indentation (use the tab key for indentation)
* and neatly written code
*/
//outputs the assignment # to the screen
cout << “This is Assignment 1A” << endl << endl;
int age;
int new_age;
cout << “Please enter your age::: ” ;
cin >> age;
new_age = age + 1;
cout << “Next year you will be ” << new_age << ” old.\n”;
cout << “Thank you for using my program!” << endl;
/*
* 1B Average of Values
* To get an average of a series of values,
* you add the values up and then divide the sum
* by the number of values.
* Write a program that stores the following values in five variables
* 28, 32, 37, 24, and 33
* the program should first calculate
* the sum of these five variables nad store the result
*in as separate variable named sum.
*Then, the program should divide the sum by 5 to get
* the average. Display the average on the screen
* TIP: Use the double data tyoe for all variables in this program
*/
//outputs the assignment # to the screen
cout << “This is Assignment 1B” << endl << endl;
//store five number variables
double num1 = 28;
double num2 = 32;
double num3 = 37;
double num4 = 24;
double num5 = 33;
//calculate the sum of the five variables and store in a variable called sum
double sum = num1 + num2 + num3 + num4 + num5;
double thisAverage = sum / 5;
cout << “thisAverage: ” << thisAverage << endl;
/*
* 1C Cyborg Data Type Sizes
* You have been given a job as a programmer on a Cyborg supercomputer.
* In order to accomplish some calculations,
* you need to know how many bytes the
* following data types use: char, int, float, and double.
* You do not have any manuals
* so you can’t look this information up.
* Write a C++ program that will determine the
* amount of memory used by these types
* and display the information to the screen.
*/
//outputs the assignment # to the screen
cout << “This is Assignment 1C” << endl << endl;
//size of char
cout << “Size of char: ” << sizeof(char) << endl;
//size of int
cout << “Size of int: ” << sizeof(int) << endl;
//size of float
cout << “Size of float: ” << sizeof(float) << endl;
//size of double
cout << “Size of double: ” << sizeof(double) << endl;
return 0;
}
SCREENSHOT OF OUTPUT:
Please note this screenshot contains output of 1A, 1B, and 1C
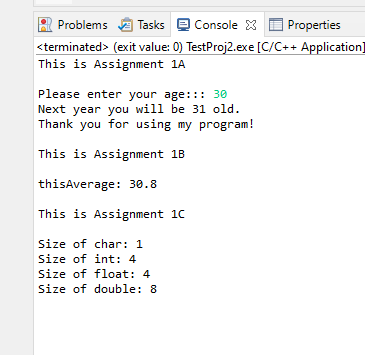
One thought on “C++ – Fortune Teller Program”