//============================================================================
// Name : MPG Application
// Description : 1A calculates the miles per gallon by taking in a car’s total // : to be driven on a full tank of gas
// : and the total gallons of gas that a car’s tank can contain
// : 1B calculates the total income generated from Class A, Class B,
// : and Class C tickets by multiplying the
// : ticket sales of each class by the number of
// : tickets sold and summing the final income amount
// : 1C is a math tutor program that randomly generates two numbers // : and sums them together, waits for a user to
// : press a key and returns the output
// Status : Complete
// Date : August 22, 2020
//============================================================================
#include <iostream>
//setprecision requires iomanip
#include <iomanip>
//rand requires cstdlib
#include <cstdlib>
using namespace std;
int main() {
/*
* Assignment 1A
* Miles per Gallon
* Write a program that calculates a car’s gas mileage.
* The program should ask the user to enter the number of gallons a car can
* hold,
* and the number of miles it can be driven on a full tank.
* It should then display the number of miles that may be driven per gallon of
* gas
*/
//Output Assignment Number
cout << “Assignment 1A” << endl << endl;
//calculate miles per gallon
//miles per gallon (mpg) =
//miles a car can be driven on a full tank / number of gallons of gas a car
// can hold
//Variable for miles driven the car can be driven on a full tank of gas
double totalMilesDriven;
//Variable for number of gallons of gas a car can hold
double totalGallonsOfGas;
//input total miles driven
cout << “Please enter the number of miles your car can be driven on a full tank of gas::: “;
cin >> totalMilesDriven;
//cout << endl;
//input total gallons of gas
cout << “Please enter the number of gallons your car’s gas tank can hold::: “;
cin >> totalGallonsOfGas;
//cout << endl;
//calculate miles per gallon
double milesPerGallon = totalMilesDriven / totalGallonsOfGas;
//output milesPerGallon
cout << “This is miles per gallon (mpg) for your car: ” << milesPerGallon << endl << endl;
/*
*Assignment 1B
*Stadium Seating
*There are three seating categories at a stadium.
*For a softball game, Class A seats cost $15, Class B seats cost $12, and
*Class C seats cost $9. Write a program that asks how many tickets for each
*seat
*were sold, then displays the amount of income generate from ticket sales.
*were Format your dollar amount in fixed-point notation, with two decimal places of
*precision, and be sure the decimal point is always displayed
*/
//Output Assignment Number
cout << “Assignment 1B” << endl << endl;
//Class A Cost
float classACost = 15.00;
//Class B Cost
float classBCost = 12.00;
//Class C Cost
float classCCost = 9.00;
//Number of Class A Tickets Sold
float numClassATickets;
//Number of Class B Tickets Sold
float numClassBTickets;
//Number of Class C Tickets Sold
float numClassCTickets;
//User Input for how many Class A tickets were sold
cout << “How many Class A Tickets were sold?: “;
cin >>numClassATickets;
//User Input for how many Class B tickets were sold
cout << “How many Class B Tickets were sold?: “;
cin >>numClassBTickets;
//User Input for how many Class C tickets were sold
cout << “How many Class C Tickets were sold?: “;
cin >>numClassCTickets;
//calculate the total tickets sold
//Total Class A Tickets Sold = Class A Price * Number of Class A Tickets Sold
float TotalClassATickets = classACost * numClassATickets;
//Total Class B Tickets Sold = Class B Price * Number of Class B Tickets Sold
float TotalClassBTickets = classBCost * numClassBTickets;
//Total Class C Tickets Sold = Class C Price * Number of Class C Tickets Sold
float TotalClassCTickets = classCCost * numClassCTickets;
//Output Class A Tickets Sold
cout << “This is the Income Generated from Total Class A Tickets Sold: $”;
cout << fixed << setprecision(2) << TotalClassATickets << endl;
//Output Class B Tickets Sold
cout << “This is the Income Generated from Total Class B Tickets Sold: $”;
cout << fixed << setprecision(2) << TotalClassBTickets << endl;
//Output Class C Tickets Sold
cout << “This is the Income Generated from Total Class C Tickets Sold: $”;
cout << fixed << setprecision(2) << TotalClassCTickets << endl;
float finIncomeTotal = TotalClassATickets + TotalClassBTickets + TotalClassCTickets;
//Output the total ticket sales
cout << “This is the total ticket sales of all Classes for the softball game: $”;
cout << fixed << setprecision(2) << finIncomeTotal << endl << endl;
/*
* Assignment 1C
* Write a program that can be used as a math tutor for a young student.
* The program should display two random numbers to be added such as:
* 247
* + 129
* The program should then pause while the student works on the program.
* When the student is ready to check the answer, he or she can press a key
* and the
* program will display the correct solution
* 247
* + 129
* ______
* 376
*/
//Output Assignment Number
cout << “Assignment 1C” << endl << endl;
int randomNumber1 = rand();
int randomNumber2 = rand();
int summedRandomNum = randomNumber1 + randomNumber2;
cout << ” ” << randomNumber1 << endl;
cout << ” + ” << randomNumber2 << endl;
cout << “————–” << endl;
cin.ignore();
cin.get();
cout << summedRandomNum << endl;
return 0;
}
Screenshots from 1A, 1B, and 1C:
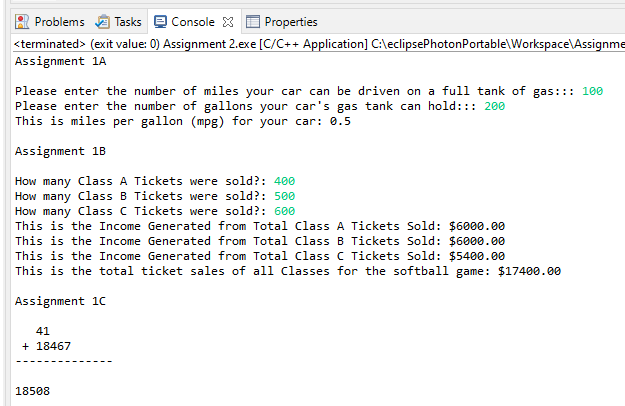
One thought on “C++ – MPG Application”