//============================================================================
// Name : Geometry Application
// Description : 3A User inputs length and width for rectangle 1 and rectangle 2
// : Then the program will output rectangle 1 and 2 area
// : Then the program will determine which area is greater or
// : equals each other
// : 3B User inputs answer to two random numbers. If answer is
// : correct then
// : message is displayed
// : otherwise the message is displayed that the answer is
// : incorrect with
// : the correct answer
// : 3C user is presented with a menu to calculate circle radius,
// : rectangle area,
// : and triangle area, or to quit the program. User selects an option
// : and inputs values
// : program returns the calculation
//
// Status : Complete
// Date : September 12 2020
//============================================================================
#include <iostream>
#include <cmath>
#include <ctime>
using namespace std;
int main() {
/*
* Assignment 3A
* Areas of a Rectangle
* The area of a rectangle is the rectangle’s length times its width
* Write a program that asks for the length and width of two rectangles
* The program should tell the user which rectangle has the greater area,
* or if the area is the same
* REMEMBER THREE SCENARIOS:
* 1. First Var > Second Var
* 2. First Var < Second Var
* 3. First Var == Second Var
*/
//Output assignment number
cout << “This is Assignment 3A:” << endl << endl;
//add efficiency to select three options
int threeACounter =0;
//since there are three scenarios loop through logic three times
while( threeACounter <3){
//post increment counter
threeACounter++;
//declare rectangle length 1
double rectangleLength1;
//declare rectangle width 1
double rectangleWidth1;
//declare rectangle length 2
double rectangleLength2;
//declare rectangle width 2
double rectangleWidth2;
//get user input for length for rectangle 1
cout << “Please input rectangle 1 length: “;
cin >> rectangleLength1;
//get user input for width for rectangle 1
cout << “Please input rectangle 1 width: “;
cin >> rectangleWidth1;
//get user input for length for rectangle 2
cout << “Please input rectangle 2 length: “;
cin >> rectangleLength2;
//get user input for width for rectangle 2
cout << “Please input rectangle 2 width: “;
cin >> rectangleWidth2;
//calculate rectangle 1 area
double rectangleArea1 = rectangleLength1 * rectangleWidth1;
//output rectangle 1 area
cout << “This is the Rectangle Area: ” << rectangleArea1 << endl;
//calculate rectangle 2 area
double rectangleArea2 = rectangleLength2 * rectangleWidth2;
//output rectangle 1 area
cout << “This is the Rectangle Area: ” << rectangleArea2 << endl;
if(rectangleArea1 > rectangleArea2){
cout << “Rectangle 1 is greater than Rectangle 2” << endl << endl;
}
else if(rectangleArea2 > rectangleArea1){
cout << “Rectangle 2 is greater than Rectangle 1” << endl << endl;
}
else if(rectangleArea1 == rectangleArea2){
cout << “Rectangle 1 equals Rectangle 2” << endl << endl;
}
}
/*
* Assignment 3B
* Modify your Assignment 2C as described below
* This is a modification of Programming Challenge 15 from Chapter 3.
* Write a program that can be used as a math tutor for a young student.
* The program should display two random numbers that are to be added such as:
* 147
* +129
* _____
* The program should wait for the student to enter the answer
* If the answer is correct, a message of congratulations should be printed
* If the answer is incorrect, a message should be printed showing the correct
* answer
*/
//Output Assignment Number
cout << “Assignment 3B” << endl << endl;
const int MIN = 50;
const int MAX = 450;
//get the system time
unsigned seed = time(0);
//see the random number generator
srand(seed);
//generate two random numbers
//two scenarios counter
int threeBCoutner = 0;
while(threeBCoutner < 2){
//post increment
threeBCoutner++;
int randomNumber1 = MIN + rand() % MAX;
int randomNumber2 = MIN + rand() % MAX;
int summedRandomNum = randomNumber1 + randomNumber2;
int yourAnswer;
cout << ” ” << randomNumber1 << endl;
cout << ” + ” << randomNumber2 << endl;
cout << “————–” << endl;
//userinput answer
cout << “Please enter your answer: ” << endl;
cin >> yourAnswer;
if(yourAnswer == summedRandomNum){
cout << “Your answer is: ” << yourAnswer <<endl;
cout << “Congratulations!!”<<endl;
}
else {
cout << “Your answer is: ” << yourAnswer << endl;
cout << “The answer is incorrect!” << endl;
cout << “This is the correct answer: ” << summedRandomNum << endl;
}
}
/*
* Assignment 3C:
* Write a program that displays the following menu:
* Geometry Calculator
* 1. Calculate the area of a circle
* 2. Calculate the area of a rectangle
* 3. Calculate the area of a triangle
* 4. Quit
*
* Enter your choice 1-4
*
* If the user enters 1 the program should ask for the radius of a circle
* and then display the area.
* area = 3.1459 * radius squared
*
* If the user enters 2 the program should ask for the length and width of the
* rectangle
* and then display the rectangle’s area. Use the following formula:
* area = length * width
*
* If the user enters 3 the program should ask for the length of the
* triangle’s base and its height,
* and then display its area. use the following formula:
* area = base * height * .5
*
* if the user enters 4 the program should end
*
* input validation: display an error message if the user enters a number
* outside of the range
* of 1 through 4 when selecting an item from the menu
* Do not accept negative values for the circle’s radius, the rectangle’s
* length or width,
* or the triangel’s base or height
*
*/
//output assignment number
cout << “\n\nThis is assignment 3C:” << endl <<endl;
//create the menu
thisStart:
string menu = “Geometry Calculator \n 1. Calculate the area of a circle \n 2. Calculate the area of a rectangle \n 3. Calculate the area of a triangle \n 4. Quit \n Enter your choice 1-4 \n”;
cout << menu;
int yourChoice;
cin >> yourChoice;
cout << endl;
if(yourChoice >=1 && yourChoice <=4){
if(yourChoice == 1){
double circleRadius;
//calculate radius of circle
//formula to calculate: area = 3.1459 * radius squared
cout << “What is the radius of the circle?” << endl;
cin >> circleRadius;
if(circleRadius > 0){
const double PI = 3.14159;
double circleArea = PI * pow(circleRadius,2.0);
cout << “This is the area of the circle: ” << circleArea << endl;
}
else{
cout << “Circle Radius cannot be less than 0.” << endl;
}
}
else if(yourChoice == 2){
//calculate rectangle
double length, width, recArea;
cout << “Please insert length: ” << endl;
cin >> length;
cout << “Please insert width: ” << endl;
cin >> width;
if(length > 0 && width > 0){
recArea = length * width;
cout << “This is the area: ” << recArea << endl;
}
else{
cout << “Rectangle length or width cannot be less than 0.” << endl;
}
}
else if(yourChoice == 3){
//calculate area of triangle
//area = base * height * .5
double base,height, triArea;
//base
cout << “Please insert the base: ” << endl;
cin >> base;
cout << “Please insert the height: ” << endl;
cin >> height;
if(base > 0 && height > 0){
triArea = base * height * .5;
cout << “This is the triangle Area: ” << triArea << endl;
}
else{
cout << “Triangle base or height cannot be less than 0.” << endl;
}
}
else if(yourChoice == 4){
//program should end
cout << “Goodbye!!”;
return 0;
}
}
else{
cout << “Invalid choice please try again!” << endl;
goto thisStart;
}
goAgainGoAgain:
char goAgain;
cout << “Do you want to go again? (y/n)” << endl;
cin >> goAgain;
if (goAgain == ‘y’){
goto thisStart;
}
else if(goAgain == ‘n’){
cout << “Goodbye!!”;
return 0;
}
else{
cout << “\nInvalid go again choice…your options are either y or n”;
goto goAgainGoAgain;
}
return 0;
}
Assignment 3 OUTPUT
3A-1. First Var > Second Var
Test Data:
#1: 30 * 30 = 900
#2: 25 * 25 = 625
3A-2. First Var < Second Var
Test Data
#1: 25 * 25 = 625
#2: 30 * 30 = 900
3A-3. First Var == Second Var
Test Data
#1: 25 * 25 = 625
#2: 25 * 25 = 625
3B-1: answer is incorrect
Input Data: any number
3B-2: answer is correct
Input Data: calculate sum on calculator to get the correct answer
3C-1-a: select option 1, then invalid negative input for radius variable
Test Data: -2
3C-2-b: select option 1, then positive input for radius
Test Data: 2
3C-2-a: select option 2, then invalid negative input for length
Test Data: Length = -1 | Width = 1
3C-2-b: select option 2, then invalid negative input for width
Test Data: Length = 1 | Width = -1
3C-2-c: select option 2, then positive input for length and width
Test Data: 2 | 2
3C-3-a: select option 3, then positive value for base, then input negative value for height
Test Data: base = 2 | height = -2
3C-3-b: select option 3, then negative value for base, then input positive value for height
Test Data: base = -2 | height = 2
3C-3-c: input positive value for height and base
Test Data: base = 2 | height = 2
3C-4-a: input number less than 1 for selection
Test Data: -1
3C-4-b: input number greater than 4 for selection
Test Data: 5
3C-5: input option 4 for goodbye
//============================================================================
// Name : Geometry Application
// Author : Zack Maher
// Description : 3A User inputs length and width for rectangle 1 and rectangle 2
// : Then the program will output rectangle 1 and 2 area
// : Then the program will determine which area is greater or
// : equals each other
// : 3B User inputs answer to two random numbers. If answer is
// : correct then
// : message is displayed
// : otherwise the message is displayed that the answer is
// : incorrect with
// : the correct answer
// : 3C user is presented with a menu to calculate circle radius,
// : rectangle area,
// : and triangle area, or to quit the program. User selects an option
// : and inputs values
// : program returns the calculation
//
// Status : Complete
// Date : September 12 2020
//============================================================================
#include <iostream>
#include <cmath>
#include <ctime>
using namespace std;
int main() {
/*
* Assignment 3A
* Areas of a Rectangle
* The area of a rectangle is the rectangle’s length times its width
* Write a program that asks for the length and width of two rectangles
* The program should tell the user which rectangle has the greater area,
* or if the area is the same
* REMEMBER THREE SCENARIOS:
* 1. First Var > Second Var
* 2. First Var < Second Var
* 3. First Var == Second Var
*/
//Output assignment number
cout << “This is Assignment 3A:” << endl << endl;
//add efficiency to select three options
int threeACounter =0;
//since there are three scenarios loop through logic three times
while( threeACounter <3){
//post increment counter
threeACounter++;
//declare rectangle length 1
double rectangleLength1;
//declare rectangle width 1
double rectangleWidth1;
//declare rectangle length 2
double rectangleLength2;
//declare rectangle width 2
double rectangleWidth2;
//get user input for length for rectangle 1
cout << “Please input rectangle 1 length: “;
cin >> rectangleLength1;
//get user input for width for rectangle 1
cout << “Please input rectangle 1 width: “;
cin >> rectangleWidth1;
//get user input for length for rectangle 2
cout << “Please input rectangle 2 length: “;
cin >> rectangleLength2;
//get user input for width for rectangle 2
cout << “Please input rectangle 2 width: “;
cin >> rectangleWidth2;
//calculate rectangle 1 area
double rectangleArea1 = rectangleLength1 * rectangleWidth1;
//output rectangle 1 area
cout << “This is the Rectangle Area: ” << rectangleArea1 << endl;
//calculate rectangle 2 area
double rectangleArea2 = rectangleLength2 * rectangleWidth2;
//output rectangle 1 area
cout << “This is the Rectangle Area: ” << rectangleArea2 << endl;
if(rectangleArea1 > rectangleArea2){
cout << “Rectangle 1 is greater than Rectangle 2” << endl << endl;
}
else if(rectangleArea2 > rectangleArea1){
cout << “Rectangle 2 is greater than Rectangle 1” << endl << endl;
}
else if(rectangleArea1 == rectangleArea2){
cout << “Rectangle 1 equals Rectangle 2” << endl << endl;
}
}
/*
* Assignment 3B
* Modify your Assignment 2C as described below
* This is a modification of Programming Challenge 15 from Chapter 3.
* Write a program that can be used as a math tutor for a young student.
* The program should display two random numbers that are to be added such as:
* 147
* +129
* _____
* The program should wait for the student to enter the answer
* If the answer is correct, a message of congratulations should be printed
* If the answer is incorrect, a message should be printed showing the correct
* answer
*/
//Output Assignment Number
cout << “Assignment 3B” << endl << endl;
const int MIN = 50;
const int MAX = 450;
//get the system time
unsigned seed = time(0);
//see the random number generator
srand(seed);
//generate two random numbers
//two scenarios counter
int threeBCoutner = 0;
while(threeBCoutner < 2){
//post increment
threeBCoutner++;
int randomNumber1 = MIN + rand() % MAX;
int randomNumber2 = MIN + rand() % MAX;
int summedRandomNum = randomNumber1 + randomNumber2;
int yourAnswer;
cout << ” ” << randomNumber1 << endl;
cout << ” + ” << randomNumber2 << endl;
cout << “————–” << endl;
//userinput answer
cout << “Please enter your answer: ” << endl;
cin >> yourAnswer;
if(yourAnswer == summedRandomNum){
cout << “Your answer is: ” << yourAnswer <<endl;
cout << “Congratulations!!”<<endl;
}
else {
cout << “Your answer is: ” << yourAnswer << endl;
cout << “The answer is incorrect!” << endl;
cout << “This is the correct answer: ” << summedRandomNum << endl;
}
}
/*
* Assignment 3C:
* Write a program that displays the following menu:
* Geometry Calculator
* 1. Calculate the area of a circle
* 2. Calculate the area of a rectangle
* 3. Calculate the area of a triangle
* 4. Quit
*
* Enter your choice 1-4
*
* If the user enters 1 the program should ask for the radius of a circle
* and then display the area.
* area = 3.1459 * radius squared
*
* If the user enters 2 the program should ask for the length and width of the
* rectangle
* and then display the rectangle’s area. Use the following formula:
* area = length * width
*
* If the user enters 3 the program should ask for the length of the
* triangle’s base and its height,
* and then display its area. use the following formula:
* area = base * height * .5
*
* if the user enters 4 the program should end
*
* input validation: display an error message if the user enters a number
* outside of the range
* of 1 through 4 when selecting an item from the menu
* Do not accept negative values for the circle’s radius, the rectangle’s
* length or width,
* or the triangel’s base or height
*
*/
//output assignment number
cout << “\n\nThis is assignment 3C:” << endl <<endl;
//create the menu
thisStart:
string menu = “Geometry Calculator \n 1. Calculate the area of a circle \n 2. Calculate the area of a rectangle \n 3. Calculate the area of a triangle \n 4. Quit \n Enter your choice 1-4 \n”;
cout << menu;
int yourChoice;
cin >> yourChoice;
cout << endl;
if(yourChoice >=1 && yourChoice <=4){
if(yourChoice == 1){
double circleRadius;
//calculate radius of circle
//formula to calculate: area = 3.1459 * radius squared
cout << “What is the radius of the circle?” << endl;
cin >> circleRadius;
if(circleRadius > 0){
const double PI = 3.14159;
double circleArea = PI * pow(circleRadius,2.0);
cout << “This is the area of the circle: ” << circleArea << endl;
}
else{
cout << “Circle Radius cannot be less than 0.” << endl;
}
}
else if(yourChoice == 2){
//calculate rectangle
double length, width, recArea;
cout << “Please insert length: ” << endl;
cin >> length;
cout << “Please insert width: ” << endl;
cin >> width;
if(length > 0 && width > 0){
recArea = length * width;
cout << “This is the area: ” << recArea << endl;
}
else{
cout << “Rectangle length or width cannot be less than 0.” << endl;
}
}
else if(yourChoice == 3){
//calculate area of triangle
//area = base * height * .5
double base,height, triArea;
//base
cout << “Please insert the base: ” << endl;
cin >> base;
cout << “Please insert the height: ” << endl;
cin >> height;
if(base > 0 && height > 0){
triArea = base * height * .5;
cout << “This is the triangle Area: ” << triArea << endl;
}
else{
cout << “Triangle base or height cannot be less than 0.” << endl;
}
}
else if(yourChoice == 4){
//program should end
cout << “Goodbye!!”;
return 0;
}
}
else{
cout << “Invalid choice please try again!” << endl;
goto thisStart;
}
goAgainGoAgain:
char goAgain;
cout << “Do you want to go again? (y/n)” << endl;
cin >> goAgain;
if (goAgain == ‘y’){
goto thisStart;
}
else if(goAgain == ‘n’){
cout << “Goodbye!!”;
return 0;
}
else{
cout << “\nInvalid go again choice…your options are either y or n”;
goto goAgainGoAgain;
}
return 0;
}
Assignment 3 OUTPUT
3A-1. First Var > Second Var
Test Data:
#1: 30 * 30 = 900
#2: 25 * 25 = 625
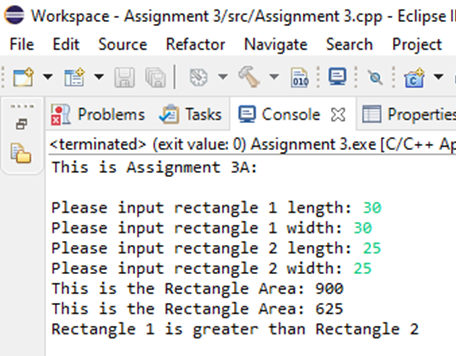
3A-2. First Var < Second Var
Test Data
#1: 25 * 25 = 625
#2: 30 * 30 = 900
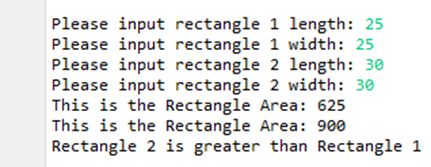
3A-3. First Var == Second Var
Test Data
#1: 25 * 25 = 625
#2: 25 * 25 = 625
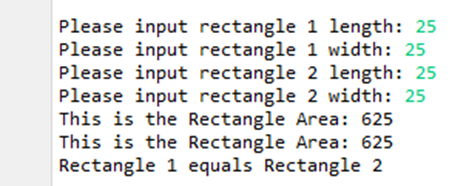
3B-1: answer is incorrect
Input Data: any number
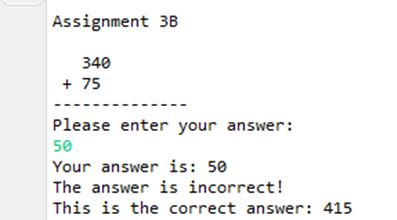
3B-2: answer is correct
Input Data: calculate sum on calculator to get the correct answer
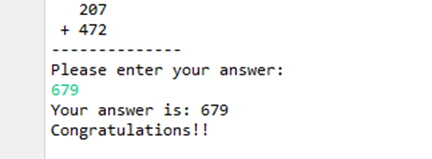
3C-1-a: select option 1, then invalid negative input for radius variable
Test Data: -2
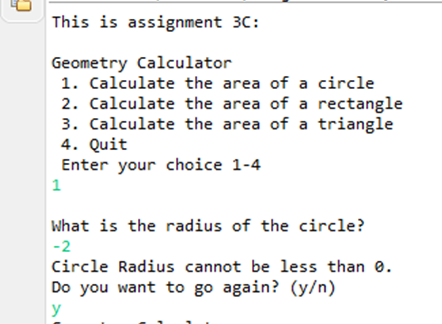
3C-2-b: select option 1, then positive input for radius
Test Data: 2
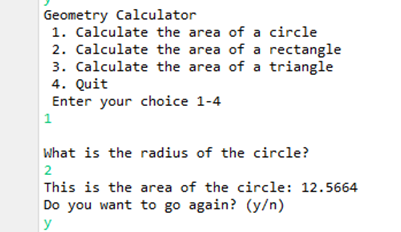
3C-2-a: select option 2, then invalid negative input for length
Test Data: Length = -1 | Width = 1
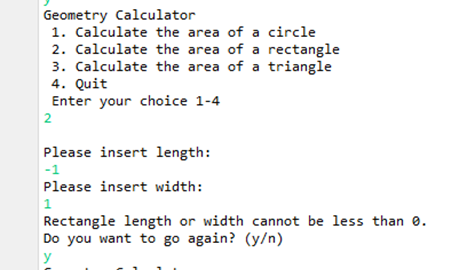
3C-2-b: select option 2, then invalid negative input for width
Test Data: Length = 1 | Width = -1
3C-2-c: select option 2, then positive input for length and width
Test Data: 2 | 2
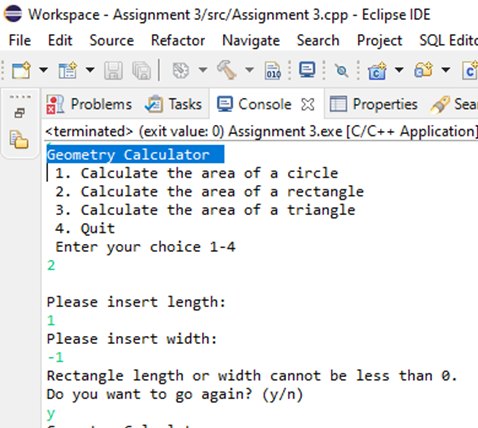
3C-3-a: select option 3, then positive value for base, then input negative value for height
Test Data: base = 2 | height = -2
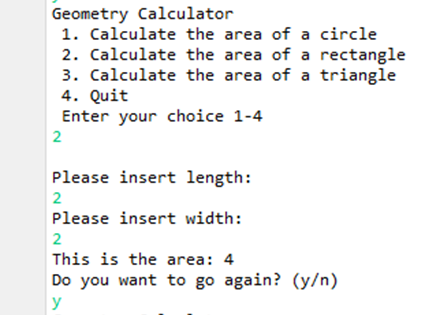
3C-3-b: select option 3, then negative value for base, then input positive value for height
Test Data: base = -2 | height = 2
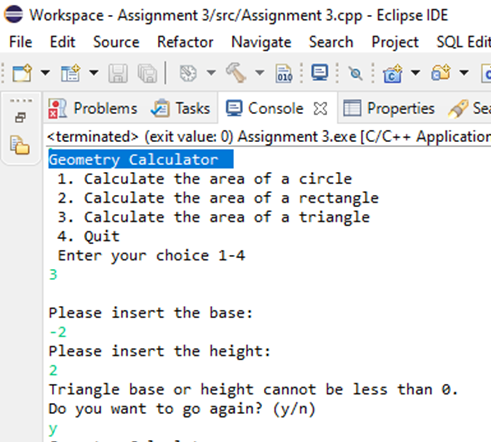
3C-3-c: input positive value for height and base
Test Data: base = 2 | height = 2
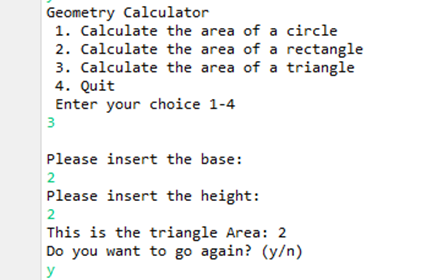
3C-4-a: input number less than 1 for selection
Test Data: -1
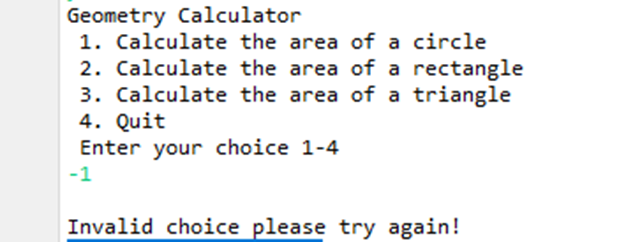
3C-4-b: input number greater than 4 for selection
Test Data: 5
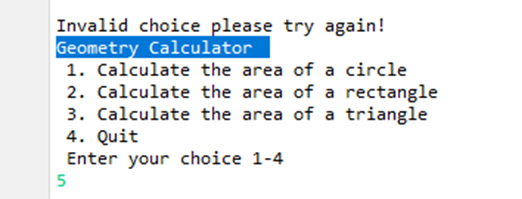
3C-5: input option 4 for goodbye
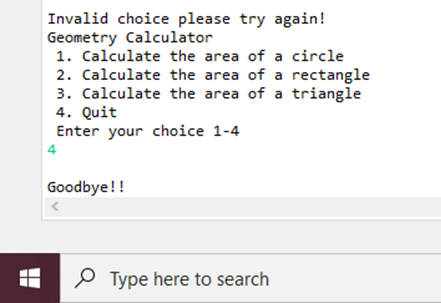
Have you ever considered publishing an e-book or guest authoring on other blogs?
I have a blog centered on the same ideas you discuss and would love to have you share some stories/information. I
know my audience would appreciate your work. If you are even remotely interested,
feel free to send me an e-mail.
I always spent my half an hour to read this weblog’s
content daily along with a cup of coffee.