//============================================================================
// Name : ASCII and Math Tutor
// Description : 4A: Displays ASCII characters
// : 4B: Math Tutor for addition, subtraction, multiplication
// : or division
// : 4C: Reads numbers from a file, calculates the number of
// : numbers
// : in the field, sum of all numbers in the file
// : The average of all numbers in the file
//============================================================================
#include <iostream>
#include <fstream>
#include <cstdlib>
#include <cmath>
#include <ctime>
using namespace std;
int main() {
/*
* 4A:
* Characters for the ASCII Codes
* Write a program that uses a loop to display the characters for the ASCII
* codes 0 through 127. Display 16 characters on each line.
*/
cout << “Start of 4a” << endl << endl;
int MINVALUE = 0;
int MAXVALUE = 127;
for(int x = MINVALUE; x <=MAXVALUE; x++){
if(x % 16 == 0){
cout << “\n”;
}
cout << char(x) << ” “;
}
cout << endl << endl <<endl << endl;
/*
* 4B:
* This program started in Programming challenge 15 of Chapter 3, and was
* modified in Programming Challenge 9 of Chapter 4. Modify the program
* again so it displays a menu allowing the user to select an addition,
* subtraction, multiplication, or division problem. The final selection on the
* menu should let the user quit the program. After the user finished the math
* problem, the program should display the menu again. This process is repeated until
* the user chooses to quit the program.
*
* Input Validation: if the use selects an item not on the menu, display an error
* message and display the menu again.
*/
cout << “Start of 4b” << endl << endl;
const int MIN = 50;
const int MAX = 540;
bool done = false;
const float SMALL = 0.00001;
double num1,num2;
double computera, usera;
int option;
string menu = “1-add, 2-subtract, 3-multiply, 4-divide, 5-exit\n\n”;
do{
cout << menu;
cin >> option;
//generate two random numbers
num1 = MIN + rand() % MAX;
num2 = MIN + rand() % MAX;
//get the system time
unsigned seed = time(0);
//seed the random number generator
srand(seed);
switch(option){
case 1:
cout << “Addition Option Started: ” << endl << endl;
computera = num1 + num2;
cout << ” ” << num1 << endl;
cout << ” + ” << num2 << endl;
cout << “————–” << endl;
//userinput answer
cout << “Please enter your answer: ” << endl;
cin >> usera;
if(usera == computera){
cout << “Your answer is: ” << usera <<endl;
cout << “Congratulations!!”<<endl;
}
else {
cout << “Your answer is: ” << usera << endl;
cout << “The answer is incorrect!” << endl;
cout << “This is the correct answer: ” << computera << endl;
}
break;
case 2:
cout << “Subtraction Process Started: ” << endl << endl;
computera = num1 – num2;
cout << ” ” << num1 << endl;
cout << ” – ” << num2 << endl;
cout << “————–” << endl;
//userinput answer
cout << “Please enter your answer: ” << endl;
cin >> usera;
if(usera == computera){
cout << “Your answer is: ” << usera <<endl;
cout << “Congratulations!!”<<endl;
}
else {
cout << “Your answer is: ” << usera << endl;
cout << “The answer is incorrect!” << endl;
cout << “This is the correct answer: ” << computera << endl;
}
break;
case 3:
cout << “Multiplication Process Started: ” << endl << endl;
computera = num1 * num2;
cout << ” ” << num1 << endl;
cout << ” * ” << num2 << endl;
cout << “————–” << endl;
//userinput answer
cout << “Please enter your answer: ” << endl;
cin >> usera;
if(usera == computera){
cout << “Your answer is: ” << usera <<endl;
cout << “Congratulations!!”<<endl;
}
else {
cout << “Your answer is: ” << usera << endl;
cout << “The answer is incorrect!” << endl;
cout << “This is the correct answer: ” << computera << endl;
}
break;
case 4:
cout << “Division Option Started: ” << endl << endl;
computera = num1 / num2;
cout << ” ” << num1 << endl;
cout << ” / ” << num2 << endl;
cout << “————–” << endl;
//userinput answer
cout << “Please enter your answer: ” << endl;
cin >> usera;
if(fabs(usera – computera) < SMALL){
cout << “Your answer is: ” << usera <<endl;
cout << “Congratulations!!”<<endl;
}
else {
cout << “Your answer is: ” << usera << endl;
cout << “The answer is incorrect!” << endl;
cout << “This is the correct answer: ” << computera << endl;
}
break;
case 5: cout << “Thank you for using my calculator” << endl;
done = true;
break;
default: cout << “Invalid choice” << endl;
}
}while(!done);
cout << endl << endl <<endl << endl;
/*
* 4C:
* Using Files – Numeric Processing
* The Student CD contains a file named random.txt. This file contains a long list of random
* numbers. Copy the file to your hard drive and then write a program that
* opens the file, reads all the numbers from the file, and calculates the following:
* A) The number of numbers in the file
* B) The sum of all the numbers in a the file (a running total)
* C) The average of all the numbers in the file
* The program should display the number of numbers found in the file, the sum of
* the numbers, and the average of the numbers.
*/
cout << “Start of 4c” << endl << endl;
//import numbers.txt
ifstream inFile;
inFile.open(“C:\\Users\\Ztmma\\Desktop\\numbers.txt”);
double num;
int numCount = 0;
double sumNum = 0;
double avgNum = 0.0;
if(!inFile){
cout << “File opening error” << endl;
exit(EXIT_FAILURE); //// you need to include the standard library for the exit function
//as follows: #include <cstdlib >
}
while(inFile >> num){
//cout << num << endl;
numCount = numCount + 1;
//cout << numCount << endl;
sumNum = sumNum + num;
//cout << sumNum << endl;
}
inFile.close();
avgNum = sumNum / numCount;
cout << “This is the count of the numbers: ” << numCount << endl;
cout << “This is the sum of the numbers: ” << sumNum << endl;
cout << “This is the average of the numbers: ” << avgNum << endl;
return 0;
Screenshot Output:
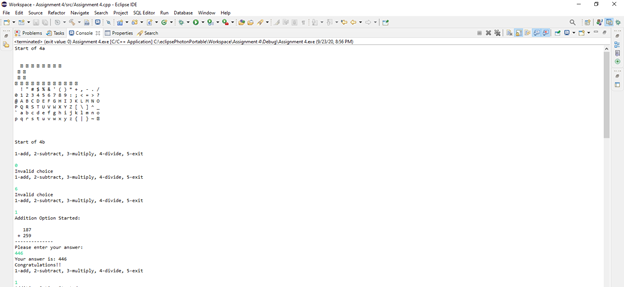
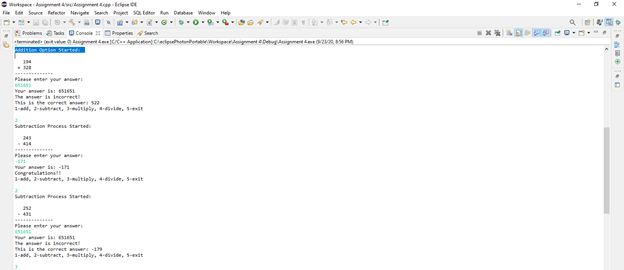
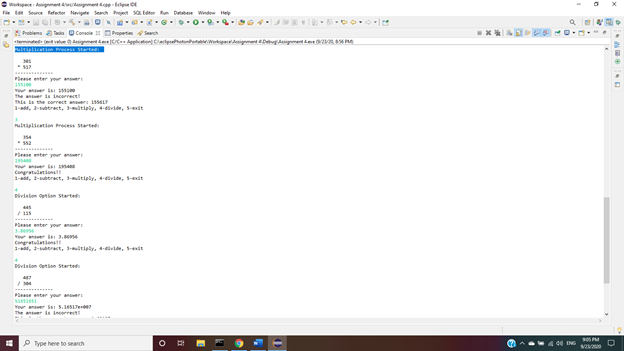
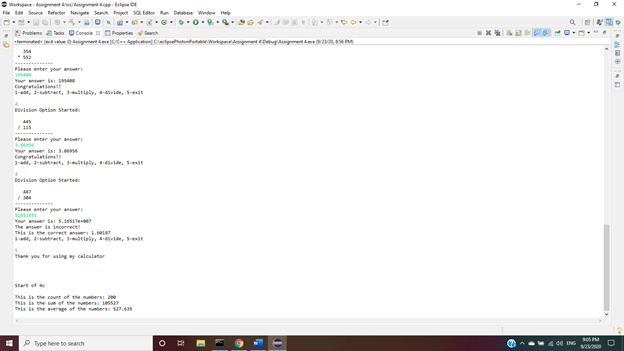
Description of output:
4a:
Screenshot for the ASCII Characters
4b-1: input value less than 1. Produce error.
4b-2: input value greater than 5. Produce error.
4b-3: sum correct answer
4b-3: sum incorrect answer
4b-4: subtraction correct answer
4b-5: subtract incorrect answer
4b-6: multiply correct answer
4b-7: multiply incorrect answer
4b-8: divide correct answer
4b-9: divide incorrect answer
4b-10: exit program
If you are going for finest contents like myself, simply go
to see this website every day because it gives quality contents, thanks
Thanks for sharing your thoughts. I truly appreciate your
efforts and I am waiting for your next write ups thanks once again.
Wow, this post is fastidious, my sister is analyzing these kinds of things, thus I am going
to convey her.